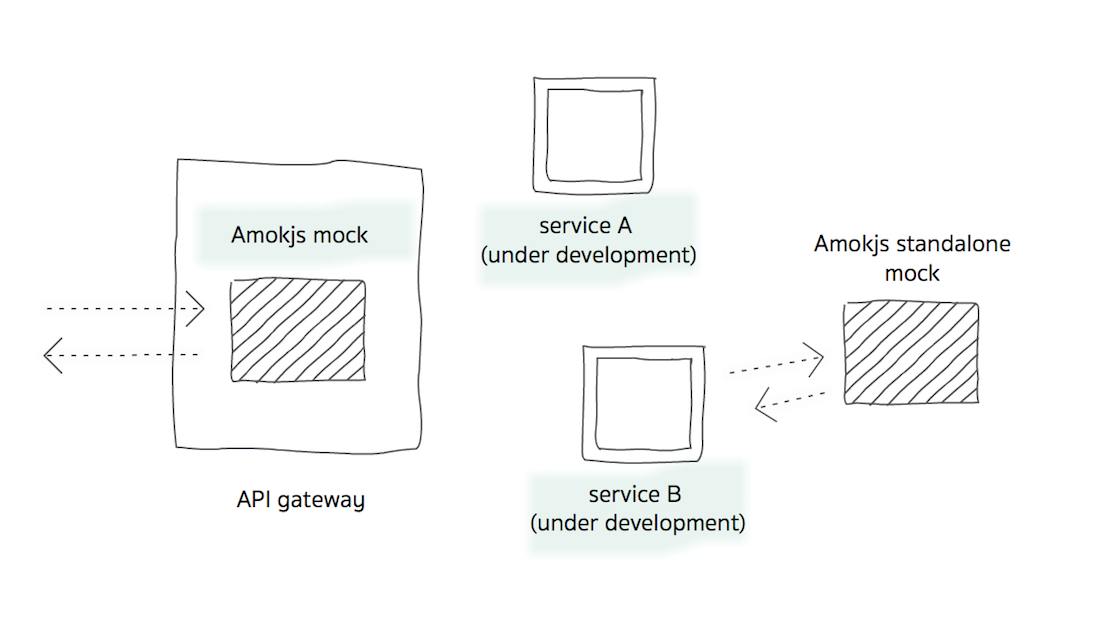
API contract mocking is often used within application development lifecycle where backend services are not yet available or not stable.
This tutorial guides you through the process of building and running simple API mocks with minimalistic Amockjs library.
We will create 2 example API mocks by using the open source API mocking framework amokjs.
One of the mocks will be lightweight and standalone Nodejs application and the second one will be Apigee bootstrap based API mock deployable on Apigee API gateway platform.
Let's get started.
Amokjs is a small Nodejs library which helps to serve example API responses from flat files.
It was created for quickly deploying API response mocks to unblock development of client applications and services.
Amokjs standalone example
First, we create the package.json
file to define Nodejs project. It must have the amokjs dependency listed.
...
"dependencies": {
"amokjs": "2.0.3"
},
...
Next, create the app.js
application file with the following
const amokjs = require('amokjs');
const port = process.env.PORT || 3000;
amokjs.setPort(port);
// start mock service
amokjs.start();
Above lines are importing amokjs package dependency and setting the incoming port on which app will listen to http requests.
What's left is to create the responses
directory and place the flat files with example responses we want to serve. Amokjs project provides few such example files as reference.
Your standalone API mock is created. Install npm dependencies and run the app
npm install
node app.js
You API mock should be serving responses at the port you specified erlier, based on the flat file name.
Let's test
curl -v localhost:3030/json
HTTP/1.1 200 OK
X-Powered-By: Express
Content-Type: text/html; charset=utf-8
Content-Length: 180
Connection: keep-alive
{
"array": [1,2,3],
"boolean": true,
"null": null,
"number": 123
}
Amokjs has few helpers. For example, support for desired http response codes via request header x-mock-response-code
and x-mock-filename
header support for removing file names from request paths.
You can find more sample responses in the Amokjs example project and start creating your own.
Apigee Edge and Amokjs Example
Apigee Edge is API management product used for building enterprise level API platforms.
We will host simple API mock using Apigee Edge and Amokjs.
For quick setup of Apigee Edge based API, we are going to use a Apigee API bootstrap framework. It has all the setup we need to get started. Follow the setup instructions for reference.
Next step is to take the standalone application we have create in the previous step of this tutorial and add it to apiproxy/resources/hosted
part of Apigee API bundle.
Finally, we have to add app.yaml
config file, which provided settings for Nodejs container within Apigee Edge.
runtime: node
runtimeVersion: 8
env:
- name: NODE_ENV
value: production
- name: LOG_LEVEL
value: 3
Running Maven deploy command again, should deploy new version of Apigee Edge API with Amokjs mock application container running.
You can find example Apigee Edge bundle with mock endpoint application on Github.